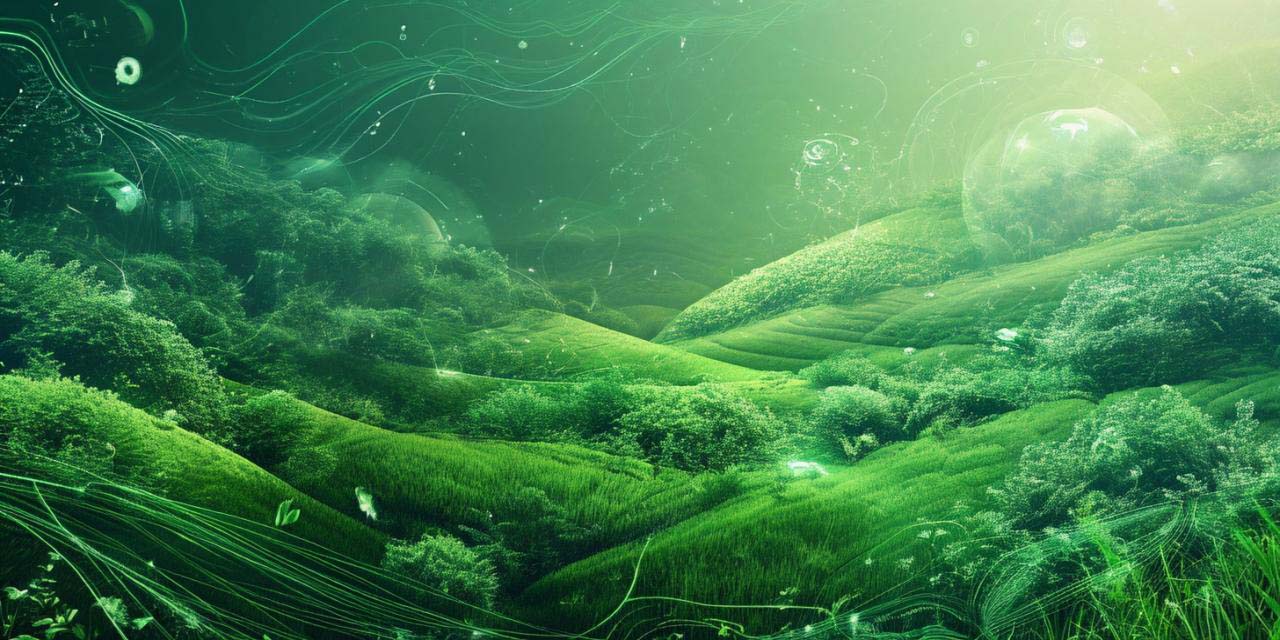
Vue3 + Vite 初级编程总结
2025年1月10日...大约 15 分钟
Vue3 + Vite 初级编程总结
基础编程
ref例子
<template>
<h1>一个人的信息</h1>
<h2>姓名:{{ name }}</h2>
<h2>年龄:{{ age }}</h2>
<h2>工作种类:{{ job.type }}</h2>
<h2>工作薪水:{{ job.salary }}</h2>
<h2>bb:{{ job.aa.bb }}</h2>
<button @click="changeInfo">修改人的信息</button>
</template>
<script>
import { ref } from 'vue'
export default {
name: 'App',
setup() {
//数据
let name = ref("张三")
let age = ref(29)
let job = ref({
type: "前端工程师",
salary: "30k",
aa: {
bb: "bb",
cc: "cc"
}
})
//方法
function changeInfo() {
name.value = "李思"
age.value = 32
job.value.type = "UI设计师"
job.value.salary = "50K"
job.value.aa.bb = "dd"
// console.log(name, age, "ylxxxxxxxxxxxxxxxxxxxx")
}
return {
age,
name,
job,
changeInfo,
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
reactive例子
<template>
<h1>一个人的信息</h1>
<h2>姓名:{{ name }}</h2>
<h2>年龄:{{ age }}</h2>
<h2>工作种类:{{ job.type }}</h2>
<h2>工作薪水:{{ job.salary }}</h2>
<h2>bb:{{ job.aa.bb }}</h2>
<h2>爱好1:{{ hobby }}</h2>
<h2>爱好2:{{ job.aa.cc.dd }}</h2>
<br>
=========================================================
<br>
<h2>姓名:{{ p.name }}</h2>
<h2>年龄:{{ p.age }}</h2>
<h2>工作种类:{{ p.job.type }}</h2>
<h2>工作薪水:{{ p.job.salary }}</h2>
<h2>爱好1:{{ p.hobby }}</h2>
<button @click="changeInfo">修改人的信息</button>
</template>
<script>
import { ref, reactive } from 'vue'
export default {
name: 'App',
setup() {
//数据=======================================================
let name = ref("张三")
let age = ref(29)
let job = reactive({
type: "前端工程师",
salary: "30k",
aa: {
bb: "bb",
cc: {
dd: ["抽烟", "喝酒", "烫头"]
}
}
})
let hobby = reactive(["抽烟", "喝酒", "烫头"])
// 定义一个对象===============================================
let person = {
name: "王五",
age: 118,
job: {
type: "后端工程师",
salary: "50K"
},
hobby: ["看电影", "搞对象", "玩游戏"]
}
let p = reactive(person)
//方法
function changeInfo() {
//响应基本数据类型--------------------------------------------
name.value = "李思"
age.value = 32
//响应object--------------------------------------------------
job.type = "UI设计师"
job.salary = "50K"
job.aa.bb = "dd"
//数组也能自动响应耶----------------------------------------------
job.aa.cc.dd[2] = "洗澡"
job.aa.cc.dd[0] = "摔跤"
hobby[1] = "学习"
hobby[0] = "运动"
//修改person对象属性值-----------------------------------------------
p.name = "互骂查"
p.age = 200
p.job.type = "无业游民"
p.job.salary = "100k"
p.hobby[0] = "编程"
}
return {
age,
name,
job,
hobby,
p,
changeInfo,
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
setup&props&context
<!-- APP.vue -->
<template>
<Demo msg="老杨同志" shcool="尚硅谷" @hello="showHelloMsg">
<template v-slot:qwe>
<span>尚硅谷好哈哈哈</span>
</template>
</Demo>
</template>
<script>
import Demo from './components/Demo.vue'
export default {
name: 'App',
components: {
Demo
},
setup() {
function showHelloMsg(value) {
alert(`你好啊,你触发了 hello 事件,我收到的参数是:${value}!`)
}
return {
showHelloMsg
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
<!-- Demo.vue -->
<template>
<h1>一个人的信息</h1>
<br>
<h2 v-show="p.name">姓名:{{ p.name }}</h2>
<h2>年龄:{{ p.age }}</h2>
<button @click="test">测试一下触发Demo组件的Hello事件</button>
</template>
<script>
import { reactive } from 'vue'
export default {
name: 'App',
props: ["msg", "shcool"],
emits:["hello"],
// slots:[],
beforeCreate() {
//console.log("------beforeCreate-----", this)
},
setup(props, context) {
//console.log("-----setup------", this, props, context)
//console.log("-----setup------", context.attrs)
//console.log("-----setup------", context.emit)
console.log("-----setup------", context.slots)
let person = {
name: "王五",
age: 118,
}
let p = reactive(person)
function test() {
context.emit('hello', "尚硅谷")
}
return {
p,
test
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
computed例子
<!-- App.vue -->
<template>
<Demo>
</Demo>
</template>
<script>
import Demo from './components/Demo.vue'
export default {
name: 'App',
components: {
Demo
},
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
<!-- Demo.vue -->
<template>
<h1>一个人的信息</h1>
<br>
姓:<input type="text" v-model="p.firstName">
<br>
名:<input type="text" v-model="p.lastName">
<br>
全名:<input type="text" v-model="p.fullName">
</template>
<script>
import { reactive, computed } from 'vue'
export default {
name: 'App',
//vue2写法
// computed: {
// fullName() {
// return this.p.firstName + "-" + this.p.lastName
// }
// },
setup() {
let person = {
firstName: "王",
lastName: "八蛋",
age: 118,
}
let p = reactive(person)
p.fullName = computed({
set(value) {
const nameArr = value.split('-')
if (nameArr.length == 2) {
p.firstName = nameArr[0]
p.lastName = nameArr[1]
}
},
get() {
return p.firstName + "-" + p.lastName
}
})
return {
p,
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
watch监视ref例子
<!-- APP.vue -->
<template>
<Demo>
</Demo>
</template>
<script>
import Demo from './components/Demo.vue'
export default {
name: 'App',
components: {
Demo
},
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
<!-- Demo.vue -->
<template>
<h1>当前求和为:{{ sum }}</h1>
<br>
<button @click="sum++">点我+1</button>
<hr>
<h1>当前的信息为:{{ msg }}</h1>
<button @click="msg += '!'">你好啊</button>
</template>
<script>
import { ref, watch } from 'vue'
export default {
name: 'App',
//vue2写法
// watch: {
// // sum(newValue, oldValue) {
// // console.log(`sum的值变化了!${newValue},${oldValue}`)
// // }
// sum: {
// immediate: true,
// deep: true,
// handler(newValue, oldValue) {
// console.log(`sum的值变化了!${newValue},${oldValue}`)
// }
// }
// },
setup() {
let sum = ref(0)
let msg = ref("你好啊")
// 监视
watch([sum, msg], (newValue, oldValue) => {
console.log(`sum的值变化了!${newValue},${oldValue}`)
}, { immediate: true, deep: true })
return {
sum,
msg
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
watch监视reactive多种情况
<template>
<Demo>
</Demo>
</template>
<script>
import Demo from './components/Demo.vue'
export default {
name: 'App',
components: {
Demo
},
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
<!-- Demo.vue -->
<template>
<h1>当前求和为:{{ sum }}</h1>
<br>
<button @click="sum++">点我+1</button>
<hr>
<h2>当前的信息为:{{ msg }}</h2>
<button @click="msg += '!'">你好啊</button>
<hr>
<h2>姓名:{{ person.name }}</h2>
<br>
<h2>年龄{{ person.age }}</h2>
<br>
<h2>薪资{{ person.job.j1.salary }}</h2>
<button @click="changeName">修改姓名</button>
<button @click="addAge">增长年龄</button>
<button @click='person.job.j1.salary++'>涨薪</button>
</template>
<script>
import { ref, reactive, watch } from 'vue'
export default {
name: 'App',
setup() {
let sum = ref(0)
let msg = ref("你好啊")
let person = reactive({
name: "张三",
age: 18,
job: {
j1: {
salary: 20
}
}
})
// 监视多个ref变量
// watch([sum, msg], (newValue, oldValue) => {
// console.log(`sum的值变化了!${newValue},${oldValue}`)
// }, { immediate: true, deep: true })
// 监视多个reactive变量,无法正确获取oldValue
// watch(person, (newValue, oldValue) => {
// console.log(`person的值变化了!`, newValue, oldValue)
// }, { immediate: true, deep: true })
// 监视某个属性,提升性能
// watch(() => person.age, (newValue, oldValue) => {
// console.log(`person的age值变化了!`, newValue, oldValue)
// }, { immediate: true, deep: true })
// 监视多个属性,提升性能
// watch([() => person.age, () => person.name], (newValue, oldValue) => {
// console.log(`person的age和name值变化了!`, newValue, oldValue)
// }, { immediate: true, deep: true })
// 监视嵌套深的属性,必须开启deep
watch(() => person.job, (newValue, oldValue) => {
console.log(`person的job值变化了!`, newValue, oldValue)
}, { immediate: true, deep: true })
function changeName() {
person.name += "~"
}
function addAge() {
person.age++
}
return {
sum,
msg,
person,
changeName,
addAge
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
watch监视ref对象例子
<!-- App.vue -->
<template>
<Demo>
</Demo>
</template>
<script>
import Demo from './components/Demo.vue'
export default {
name: 'App',
components: {
Demo
},
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
<!-- Demo.vue -->
<template>
<h1>当前求和为:{{ sum }}</h1>
<br>
<button @click="sum++">点我+1</button>
<hr>
<h2>当前的信息为:{{ msg }}</h2>
<button @click="msg += '!'">你好啊</button>
<hr>
<h2>姓名:{{ person.name }}</h2>
<br>
<h2>年龄{{ person.age }}</h2>
<br>
<h2>薪资{{ person.job.j1.salary }}</h2>
<button @click="person.name += '~'">修改姓名</button>
<button @click="person.age++">增长年龄</button>
<button @click='person.job.j1.salary++'>涨薪</button>
</template>
<script>
import { ref, watch } from 'vue'
export default {
name: 'App',
setup() {
let sum = ref(0)
let msg = ref("你好啊")
let person = ref({
name: "张三",
age: 18,
job: {
j1: {
salary: 20
}
}
})
//ref person 对象时监视用法。需要给对象加value
// watch(person.value, (newValue, oldValue) => {
// console.log(newValue, oldValue)
// })
//ref person 对象时监视用法。需要给对象加value
watch(person, (newValue, oldValue) => {
console.log(newValue, oldValue)
}, { deep: true })
return {
sum,
msg,
person,
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
watchEffect例子
<!-- App.vue -->
<template>
<Demo>
</Demo>
</template>
<script>
import Demo from './components/Demo.vue'
export default {
name: 'App',
components: {
Demo
},
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
<!-- Demo.vue -->
<template>
<h1>当前求和为:{{ sum }}</h1>
<br>
<button @click="sum++">点我+1</button>
<hr>
<h2>当前的信息为:{{ msg }}</h2>
<button @click="msg += '!'">你好啊</button>
<hr>
<h2>姓名:{{ person.name }}</h2>
<br>
<h2>年龄{{ person.age }}</h2>
<br>
<h2>薪资{{ person.job.j1.salary }}</h2>
<button @click="person.name += '~'">修改姓名</button>
<button @click="person.age++">增长年龄</button>
<button @click='person.job.j1.salary++'>涨薪</button>
</template>
<script>
import { ref, reactive, watch, watchEffect } from 'vue'
export default {
name: 'App',
setup() {
let sum = ref(0)
let msg = ref("你好啊")
let person = reactive({
name: "张三",
age: 18,
job: {
j1: {
salary: 20
}
}
})
// watch(sum, (newValue, oldValue) => {
// console.log(newValue, oldValue)
// }, { immediate: true, deep: true })
// 函数里面用到的变量都会自动监视
watchEffect(() => {
const x1 = sum.value
const x2 = person.job.j1.salary
console.log("watchEffect 所指定的回调执行了")
})
return {
sum,
msg,
person,
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
生命周期钩子
<!-- App.vue -->
<template>
<button @click="isShowDemo=!isShowDemo">切换隐藏/显示</button>
<br>
<Demo v-if="isShowDemo"></Demo>
</template>
<script>
import Demo from './components/Demo.vue'
import {ref} from 'vue'
export default {
name: 'App',
components: {
Demo
},
setup(){
let isShowDemo= ref(true)
return {
isShowDemo,
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
<!-- Demo.vue -->
<template>
<h1>当前求和为:{{ sum }}</h1>
<br>
<button @click="sum++">点我+1</button>
</template>
<script>
import { ref, onBeforeMount, onMounted, onBeforeUpdate, onUpdated, onBeforeUnmount, onUnmounted } from 'vue'
export default {
name: 'App',
setup() {
console.log("---setup-等价于beforeCreate和create------")
let sum = ref(0)
onBeforeMount(() => {
console.log("---onBeforeMount-------")
})
onMounted(() => {
console.log("---onMounted-------")
})
onBeforeUpdate(() => {
console.log("---onBeforeUpdate-------")
})
onUpdated(() => {
console.log("---onUpdated-------")
})
onBeforeUnmount(() => {
console.log("---onBeforeUnmount-------")
})
onUnmounted(() => {
console.log("---onUnmounted-------")
})
return {
sum,
}
},
//通过配置项来测试生命周期
// beforeCreate(){
// console.log("---beforeCreate-------")
// },
// create(){
// console.log("---create-------")
// },
// beforeMount(){
// console.log("---beforeMount-------")
// },
// mounted(){
// console.log("---mounted-------")
// },
// beforeUpdate(){
// console.log("---beforeUpdate-------")
// },
// updated(){
// console.log("---updated-------")
// },
// beforeUnmount(){
// console.log("---beforeUnmount-------")
// },
// unmounted(){
// console.log("---unmounted-------")
// }
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
hooks例子
<!-- App.vue -->
<template>
<button @click="isShowDemo=!isShowDemo">切换隐藏/显示</button>
<br>
<Demo v-if="isShowDemo"></Demo>
<hr>
<Test></Test>
</template>
<script>
import Demo from './components/Demo.vue'
import Test from './components/Test.vue'
import {ref} from 'vue'
export default {
name: 'App',
components: {
Demo,
Test
},
setup(){
let isShowDemo= ref(true)
return {
isShowDemo,
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
<!-- Test.vue -->
<template>
<h1>我是Test组件... ... ...</h1>
<h1>点击时鼠标的坐标是:{{ point.x }},{{ point.y }}</h1>
</template>
<script>
import usePoint from '../hooks/usePoint'
export default {
name: 'Test-Component',
setup() {
const point = usePoint()
return {
point
}
},
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
<!-- Demo.vue -->
<template>
<h1>当前求和为:{{ sum }}</h1>
<br>
<button @click="sum++">点我+1</button>
<hr>
<h1>点击时鼠标的坐标是:{{ point.x }},{{ point.y }}</h1>
</template>
<script>
import { ref, } from 'vue'
import usePoint from '../hooks/usePoint'
export default {
name: 'Demo-Component',
setup() {
let sum = ref(0)
const point = usePoint()
return {
sum,
point
}
},
//通过配置项来测试生命周期
// beforeCreate(){
// console.log("---beforeCreate-------")
// },
// create(){
// console.log("---create-------")
// },
// beforeMount(){
// console.log("---beforeMount-------")
// },
// mounted(){
// console.log("---mounted-------")
// },
// beforeUpdate(){
// console.log("---beforeUpdate-------")
// },
// updated(){
// console.log("---updated-------")
// },
// beforeUnmount(){
// console.log("---beforeUnmount-------")
// },
// unmounted(){
// console.log("---unmounted-------")
// }
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
// hooks/usePoints.js
import { onMounted, reactive, onBeforeUnmount} from 'vue'
export default function(){
let point = reactive({
x: 0,
y: 0
})
function savePoint(event) {
point.x = event.pageX
point.y = event.pageY
console.log(event.pageX, event.pageY)
}
onMounted(() => {
window.addEventListener('click', savePoint)
})
onBeforeUnmount(() => {
window.removeEventListener('click', savePoint)
})
return point
}
toRef和toRefs例子
<!-- App.vue -->
<template>
<button @click="isShowDemo=!isShowDemo">切换隐藏/显示</button>
<br>
<Demo v-if="isShowDemo"></Demo>
<hr>
</template>
<script>
import Demo from './components/Demo.vue'
import {ref} from 'vue'
export default {
name: 'App',
components: {
Demo,
},
setup(){
let isShowDemo= ref(true)
return {
isShowDemo,
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
<!-- Demo.vue -->
<template>
<h2>全部信息:{{ person }}</h2>
<h2>姓名:{{ name }}</h2>
<br>
<h2>年龄{{ age }}</h2>
<br>
<h2>薪资{{ job.j1.salary }}</h2>
<button @click="name += '~'">修改姓名</button>
<button @click="age++">增长年龄</button>
<button @click='job.j1.salary++'>涨薪</button>
</template>
<script>
import { reactive, toRef, toRefs } from 'vue'
export default {
name: 'App',
setup() {
let person = reactive({
name: "张三",
age: 18,
job: {
j1: {
salary: 20
}
}
})
return {
person,
...toRefs(person),
// name: toRef(person, "name"),
// age: toRef(person, "age"),
// salary: toRef(person.job.j1, "salary"),
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
shallowRef和shallowReactive例子
<!-- App.vue -->
<template>
<button @click="isShowDemo=!isShowDemo">切换隐藏/显示</button>
<br>
<Demo v-if="isShowDemo"></Demo>
<hr>
</template>
<script>
import Demo from './components/Demo.vue'
import {ref} from 'vue'
export default {
name: 'App',
components: {
Demo,
},
setup(){
let isShowDemo= ref(true)
return {
isShowDemo,
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
<!-- Demo.vue -->
<template>
<h2>x:{{ x.y }}</h2>
<h2>全部:{{ person }}</h2>
<h2>姓名:{{ name }}</h2>
<br>
<h2>年龄{{ age }}</h2>
<br>
<h2>薪资{{ job.j1.salary }}</h2>
<button @click="name += '~'">修改姓名</button>
<button @click="age++">增长年龄</button>
<button @click='job.j1.salary++'>涨薪</button>
<button @click='(x.y)++'>x+++++</button>
</template>
<script>
import { reactive, ref, toRefs, shallowReactive, shallowRef } from 'vue'
export default {
name: 'App',
setup() {
let person = shallowReactive({
name: "张三",
age: 18,
job: {
j1: {
salary: 20
}
}
})
let x = ref({
y: 0
})
// let x = shallowRef({
// y: 0
// })
return {
x,
person,
...toRefs(person),
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
shallowReadonly和readOnly例子
<!-- App.vue -->
<template>
<button @click="isShowDemo=!isShowDemo">切换隐藏/显示</button>
<br>
<Demo v-if="isShowDemo"></Demo>
<hr>
</template>
<script>
import Demo from './components/Demo.vue'
import {ref} from 'vue'
export default {
name: 'App',
components: {
Demo,
},
setup(){
let isShowDemo= ref(true)
return {
isShowDemo,
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
<!-- Demo.vue -->
<template>
<h2>x:{{ x }}</h2>
<h2>姓名:{{ name }}</h2>
<br>
<h2>年龄{{ age }}</h2>
<br>
<h2>薪资{{ job.j1.salary }}</h2>
<button @click="name += '~'">修改姓名</button>
<button @click="age++">增长年龄</button>
<button @click='job.j1.salary++'>涨薪</button>
<button @click='x++'>x+++++</button>
</template>
<script>
import { reactive, ref, toRefs, readonly, shallowReadonly } from 'vue'
export default {
name: 'App',
setup() {
let person = reactive({
name: "张三",
age: 18,
job: {
j1: {
salary: 20
}
}
})
let x = ref(0)
// person = readonly(person)
// x = shallowReadonly(x)
person = shallowReadonly(person)
x = readonly(x)
return {
x,
...toRefs(person),
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
raw与markRaw例子
<!-- App.vue -->
<template>
<button @click="isShowDemo=!isShowDemo">切换隐藏/显示</button>
<br>
<Demo v-if="isShowDemo"></Demo>
<hr>
</template>
<script>
import Demo from './components/Demo.vue'
import {ref} from 'vue'
export default {
name: 'App',
components: {
Demo,
},
setup(){
let isShowDemo= ref(true)
return {
isShowDemo,
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
<!-- Demo.vue -->
<template>
<h2>x:{{ x }}</h2>
<h2>姓名:{{ name }}</h2>
<br>
<h2>年龄{{ age }}</h2>
<br>
<h2>薪资{{ job.j1.salary }}</h2>
<button @click="name += '~'">修改姓名</button>
<button @click="age++">增长年龄</button>
<button @click='job.j1.salary++'>涨薪</button>
<button @click='x++'>x+++++</button>
<button @click='showRaw'>输出最原始的person</button>
<br>
<h2 v-show="person.car">汽车{{ person.car }}</h2>
<button @click='addCar'>给人添加一台车</button>
<button v-show="person.car" @click='person.car.name +="~"'>换车名</button>
<button v-show="person.car" @click='person.car.price++'>换价格</button>
</template>
<script>
import { reactive, ref, toRaw, toRefs, markRaw } from 'vue'
export default {
name: 'App',
setup() {
let person = reactive({
name: "张三",
age: 18,
job: {
j1: {
salary: 20
}
}
})
let x = ref(0)
function showRaw() {
const p = toRaw(person)
console.log(p)
// ref 对象不能使用
// const sum = toRaw(x)
// console.log(sum)
}
function addCar() {
const car = { name: "奔驰", price: 40 }
person.car = markRaw(car)
console.log(person.car)
}
return {
x,
person,
...toRefs(person),
showRaw,
addCar
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
customRef例子
<!-- App.vue -->
<template>
<input type="text" v-model="keyWord">
<h3>{{ keyWord }}</h3>
</template>
<script>
import { ref, customRef } from 'vue'
export default {
name: 'App',
setup() {
//let keyWord = ref('hello')//使用 vue 提供的 ref 来实现实时动态更新功能
function myRef(value,delay) {
let timer
console.log("自定义的myRef", value)
return customRef((track, trigger) => {
return {
get() {
console.log("有人从myRef中读取数据了", value)
track() //通知 vue 追踪 value
return value
},
set(newValue) {
console.log("有人改数据了", newValue)
value = newValue
clearTimeout(timer)
timer = setTimeout(() => {
trigger() //通知 vue 重新解析模板
}, delay);
}
}
})
}
let keyWord = myRef('hello',500)//使用自定义的 ref 来实现实时动态更新功能
return {
keyWord,
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
provide和inject例子
<!-- App.vue -->
<template>
<div class="app">
<h3>我是APP组件 祖 {{ name }}----{{ price }}</h3>
<Child />
</div>
</template>
<script>
import { reactive, toRefs, provide } from 'vue';
import Child from './components/Child.vue';
export default {
name: 'App',
components: { Child },
setup() {
let car = reactive({
name: "奔驰",
price: 40
})
provide("car", car)//给自己的后代组件传递数据
return {
...toRefs(car)
}
}
}
</script>
<style>
.app {
background-color: blueviolet;
padding: 10px;
}
</style>
<!-- child.vue -->
<template>
<div class="child">
<h3>我是child组件 子</h3>
<Son />
</div>
</template>
<script>
import Son from "./Son.vue"
export default {
name: 'Child-c',
components: { Son },
setup() {
}
}
</script>
<style>
.child {
background-color: rgb(58, 226, 43);
padding: 10px;
}
</style>
<!-- son.vue -->
<template>
<div class="son">
<h3>我是Son组件 孙 {{ name }}--vvvvv---{{ price }} www</h3>
</div>
</template>
<script>
import { inject, toRefs } from 'vue';
export default {
name: 'Son-c',
setup() {
let car = inject('car')
console.log(car, "son inject拿到数据了")
return {
...toRefs(car)
}
}
}
</script>
<style>
.son {
background-color: rgb(226, 43, 58);
padding: 10px;
}
</style>
isRef等判断语法例子
<!-- App.vue -->
<template>
<div class="app">
<h3>我是APP组件 祖 {{ name }}----{{ price }}</h3>
</div>
</template>
<script>
import { ref, reactive, toRefs, readonly, isRef, isReactive, isReadonly, isProxy } from 'vue';
export default {
name: 'App',
setup() {
let car = reactive({
name: "奔驰",
price: 40
})
let sum = ref(0)
let car2 = readonly(car)
console.log(isRef(sum))
console.log(isReactive(car))
console.log(isReadonly(car2))
console.log(isProxy(car))
console.log(isProxy(car2))
return {
...toRefs(car)
}
}
}
</script>
<style>
.app {
background-color: blueviolet;
padding: 10px;
}
</style>
telerport例子
<!-- App.vue -->
<template>
<div class="app">
<h3>我是APP组件</h3>
<Child />
</div>
</template>
<script>
import Child from './components/Child.vue';
export default {
name: 'App',
components: { Child },
}
</script>
<style>
.app {
background-color: blueviolet;
padding: 10px;
}
</style>
<!-- child.vue -->
<template>
<div class="child">
<h3>我是child组件</h3>
<Son />
</div>
</template>
<script>
import Son from "./Son.vue"
export default {
name: 'Child-c',
components: { Son },
}
</script>
<style>
.child {
background-color: rgb(58, 226, 43);
padding: 10px;
}
</style>
<!-- Son.vue -->
<template>
<div class="son">
<h3>我是Son组件</h3>
<Dialog></Dialog>
</div>
</template>
<script>
import Dialog from './Dialog.vue';
export default {
name: 'Son-c',
components: { Dialog }
}
</script>
<style>
.son {
background-color: rgb(226, 43, 58);
padding: 10px;
}
</style>
<!-- Dialouge.vue -->
<template>
<div>
<button @click="isShow = true">点我谈个窗</button>
<Teleport to="body">
<div class="mask" v-if="isShow">
<div class="dialouge">
<h3>我是一个弹窗</h3>
<h4>一些数据内容呢</h4>
<h4>一些数据内容呢</h4>
<h4>一些数据内容呢</h4>
<button @click="isShow = false">关闭弹窗</button>
</div>
</div>
</Teleport>
</div>
</template>
<script>
import { ref } from "vue"
export default {
name: 'Dialogue-c',
setup() {
let isShow = ref(false)
return {
isShow
}
}
}
</script>
<style>
.dialouge {
background-color: rgb(43, 144, 226);
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
padding: 10px;
width: 300px;
height: 300px;
text-align: center;
}
.mask {
position: absolute;
top: 0;
bottom: 0;
left: 0;
right: 0;
background-color: rgba(0, 0, 0, 0.5);
}
</style>
Suspense例子
<!-- App.vue -->
<template>
<div class="app">
<h3>我是APP组件</h3>
<Suspense>
<template v-slot:default>
<Child />
</template>
<template v-slot:fallback>
<h3>稍等,加载中... ...</h3>
</template>
</Suspense>
</div>
</template>
<script>
// import Child from './components/Child.vue';
import { defineAsyncComponent } from 'vue';//静态引入
const Child = defineAsyncComponent(() => { //异步引入
return import("./components/Child.vue")
})
export default {
name: 'App',
components: { Child },
}
</script>
<style>
.app {
background-color: blueviolet;
padding: 10px;
}
</style>
<!-- Child.vue -->
<template>
<div class="child">
<h3>我是child组件</h3>
{{ sum }}
</div>
</template>
<script>
import { ref } from 'vue'
export default {
name: 'Child-c',
async setup() {
let sum = ref(0)
let p = new Promise((resolve, reject) => {
setTimeout(() => {
resolve({ sum })
}, 5000);
})
return await p
}
}
</script>
<style>
.child {
background-color: rgb(58, 226, 43);
padding: 10px;
}
</style>